Feature Scaling: A Method to Maximize the Potential of Data
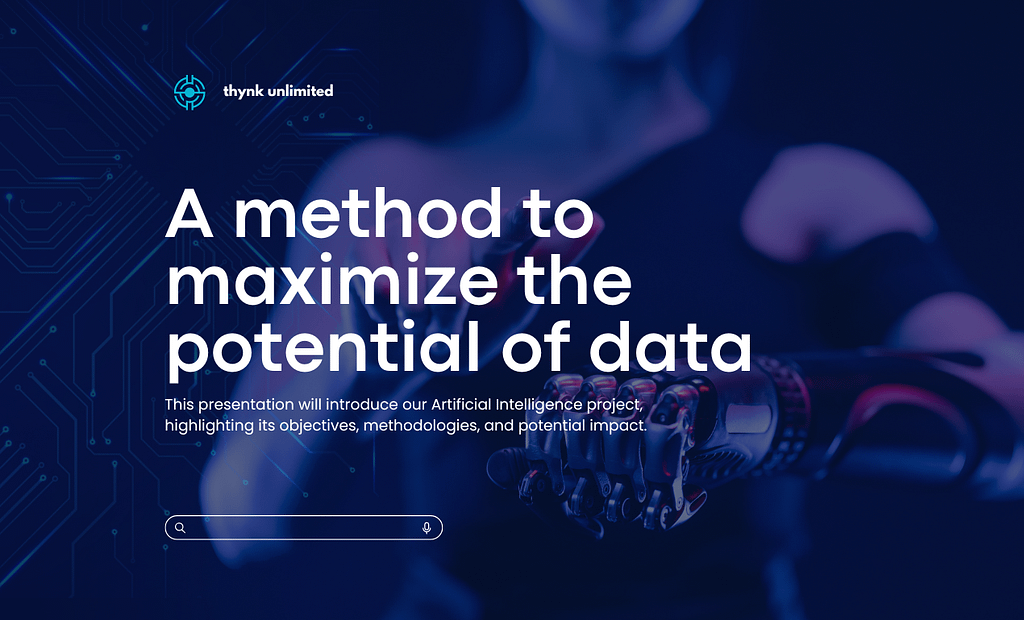
In the realm of data science and machine learning, the preprocessing of data plays a crucial role in the performance and accuracy of models. Among the various preprocessing techniques, feature scaling stands out as a fundamental method to enhance the effectiveness of algorithms. This blog delves into the concept of feature scaling, its importance, different methods, and practical applications in maximizing the potential of data.
What is Feature Scaling?
Feature scaling is the process of transforming the values of features within a dataset to a common scale, typically without distorting the differences in the ranges of values. This ensures that no single feature dominates the learning process due to its magnitude. Feature scaling is essential when features in a dataset vary widely in terms of units or ranges.
Why is Feature Scaling Important?
- Improves Model Performance:
- Many machine learning algorithms, especially those that use distance metrics like k-nearest neighbors (KNN) and support vector machines (SVM), are sensitive to the magnitude of features. Scaling ensures that all features contribute equally to the model’s predictions.
- Accelerates Convergence in Gradient Descent:
- Algorithms that rely on gradient descent optimization, such as linear regression and neural networks, benefit significantly from feature scaling. It helps in achieving faster convergence by preventing features with larger values from dominating the updates.
- Enhances Interpretability:
- Scaling data makes it easier to compare and interpret the significance of different features. It standardizes the range of values, making the model’s coefficients more interpretable.
- Prevents Numerical Instability:
- Large differences in feature values can lead to numerical instability in algorithms. Feature scaling mitigates this risk by normalizing the ranges of values.
Common Methods of Feature Scaling
- Min-Max Scaling (Normalization):
- This technique rescales the feature values to a specific range, usually [0, 1] or [-1, 1].
from sklearn.preprocessing import MinMaxScaler
scaler = MinMaxScaler()
scaled_data = scaler.fit_transform(data)
- Standardization (Z-score Normalization):
- Standardization transforms the data to have a mean of zero and a standard deviation of one.
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
scaled_data = scaler.fit_transform(data)
- Robust Scaling:
- This method uses the median and interquartile range to scale the data, making it robust to outliers.
from sklearn.preprocessing import RobustScaler
scaler = RobustScaler()
scaled_data = scaler.fit_transform(data)
- MaxAbs Scaling:
- This technique scales the data by its maximum absolute value, preserving the sparsity of the dataset.
from sklearn.preprocessing import MaxAbsScaler
scaler = MaxAbsScaler()
scaled_data = scaler.fit_transform(data)
Practical Applications of Feature Scaling
- Machine Learning Algorithms:
- Algorithms such as KNN, SVM, and principal component analysis (PCA) perform better with scaled data. For instance, in KNN, distances between data points are more accurately represented when features are scaled.
- Neural Networks:
- Feature scaling is crucial for the efficient training of neural networks. It ensures that the gradients are well-behaved, leading to faster and more stable training processes.
- Clustering Algorithms:
- Clustering algorithms like k-means and hierarchical clustering use distance measures to form clusters. Scaled features ensure that each feature contributes equally to the distance calculations.
- Time Series Analysis:
- In time series forecasting, scaling can improve the performance of models like ARIMA and LSTM by ensuring that features are on comparable scales.
Case Study: Impact of Feature Scaling on Model Performance
Consider a dataset with features representing the height (in centimeters) and weight (in kilograms) of individuals. Without scaling, the weight feature may dominate due to its larger range of values, leading to biased model predictions. By applying feature scaling, both features are brought to a comparable scale, resulting in improved model accuracy and interpretability.
import pandas as pd
from sklearn.preprocessing import StandardScaler
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Sample data
data = {'Height': [150, 160, 170, 180, 190],
'Weight': [65, 70, 75, 80, 85],
'Age': [25, 35, 45, 55, 65]}
df = pd.DataFrame(data)
# Features and target
X = df[['Height', 'Weight']]
y = df['Age']
# Train-test split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Without scaling
model = LinearRegression()
model.fit(X_train, y_train)
predictions = model.predict(X_test)
print("MSE without scaling:", mean_squared_error(y_test, predictions))
# With scaling
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
model.fit(X_train_scaled, y_train)
predictions = model.predict(X_test_scaled)
print("MSE with scaling:", mean_squared_error(y_test, predictions))
In this example, applying standard scaling significantly reduces the mean squared error (MSE), demonstrating the importance of feature scaling in improving model performance.
Table of Contents
Conclusion
Feature scaling is a vital preprocessing step in machine learning that ensures all features contribute equally to the model’s predictions. By transforming feature values to a common scale, it enhances the performance, stability, and interpretability of models across various applications. Whether you’re working with distance-based algorithms, gradient descent optimization, or neural networks, incorporating feature scaling into your data preprocessing pipeline is essential for maximizing the potential of your data.